E2E Testing
Understanding and implementing End-to-End (E2E) testing in software development
2 minute read
End-to-end tests validate the entire software system, including its integration with external interfaces. They exercise complete production-like scenarios, typically executed after functional testing.
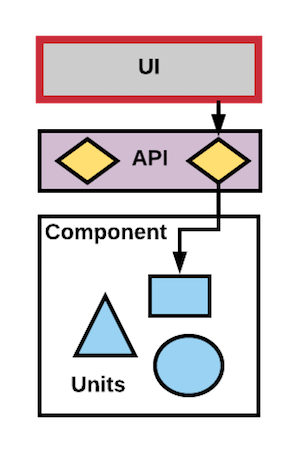
E2E Test
Types of E2E Tests
Vertical E2E Tests
Target features under the control of a single team. Examples:
- Favoriting an item and persisting across refresh
- Creating a new saved list and adding items to it
Horizontal E2E Tests
Span multiple teams. Example:
- Going from homepage through checkout (involves homepage, item page, cart, and checkout teams)
Note
Due to their complexity, horizontal tests are unsuitable for blocking release pipelines.Recommended Best Practices
E2E tests should be the least used due to their cost in run time and in maintenance required.
- Focus on happy-path validation of business flows
- E2E tests can fail for reasons unrelated to the coding issues. Capture the frequency and cause of failures so that efforts can be made to make them more stable.
- Vertical E2E tests should be maintained by the team at the start of the flow and versioned with the component (UI or service).
- CD pipelines should be optimized for the rapid recovery of production issues. Therefore, horizontal E2E tests should not be used to block delivery due to their size and relative failure surface area.
- A team may choose to run vertical E2E in their pipeline to block delivery, but efforts must be made to decrease false positives to make this valuable.
Alternate Terms
“Integration test” and “end-to-end test” are often used interchangeably.
Resources
- Testing Strategies in a Microservice Architecture: E2E Introduction
- The Practical Test Pyramid: E2E Tests
- Google Test Blog: Just Say No to More End-to-End Tests